- Microsoft Visual Studio Samples
- Visual Studio Force Formatting
- Microsoft Visual Studio Guide
- Visual Studio Formatting Settings
- Visual Studio 2017 Formatting
Visual Studio Code has built in formatters for TypeScript, C# and Go, but I want formatters for html, scss and javascript as well. Visual Studio Code provides a formatting API, so other developers can create formatters for programming languages. There is a great blog article on how to write a. Formatting code You can use Visual Studio to automatically format your code so that your code is written consistently. The following two commands explicitly format your code: Format Document (Edit → Advanced → Format Document, or Ctrl + K, then Ctrl + D) – Formats the entire active file.
Default Formatter
Code formatting is supported using either one of yapf or autopep8.
Install catalina terminal. The default code format provider is autopep8.
Auto Formatting
Formatting the source code as and when you save the contents of the file is supported.
Enabling this requires configuring the setting 'editor.formatOnSave': true
as identified here.
Paths
All samples provided here are for windows.
However Mac/Linux paths are also supported.
AutoPep8
You can configure the format provider by changing a setting in the User or Workspace settings file as follows:
Installing autopep8
Custom Path
This is generally unnecessary. As the Extension will resolve the path to the formatter based on Python executable being used or configured in python.pythonPath of settings.json. If this cannot be found, then the formatter will be resolved based on the current environment Path settings.
If this is not the case or you wish to use another version of autopep8, all you need to do is configure the path as follows either in the User or Workspace settings file:
Yapf
You can configure the format provider by changing a setting in the User or Workspace settings file as follows:
Custom Path
This is generally unnecessary. As the Extension will resolve the path to the formatter based on Python executable being used or configured in python.pythonPath of settings.json. If this cannot be found, then the formatter will be resolved based on the current environment Path settings.
If this is not the case or you wish to use another version of yapf, all you need to do is configure the path as follows either in the User or Workspace settings file:
Custom arguments to Yapf
Custom arguments can be passed into yaps by configuring the settings in the User or Workspace settings as follows:
Installing Yapf
pip install yapf
-->Formatting rules affect how indentation, spaces, and new lines are aligned around .NET programming language constructs. The rules fall into the following categories:
- .NET formatting rules: Rules that apply to both C# and Visual Basic. The EditorConfig option names for these rules start with
dotnet_
prefix. - C# formatting rules: Rules that are specific to C# language only. The EditorConfig option names for these rules start with
csharp_
prefix.
Rule ID: 'IDE0055' (Fix formatting)
All formatting options have rule ID IDE0055
and title Fix formatting
. Set the severity of a formatting violation in an EditorConfig file using the following configuration line.
The severity value must be warning
or error
to be enforced on build. For all possible severity values, see severity level.
Option format
Options for formatting rules can be specified in an EditorConfig file with the following format:
rule_name = value
For many rules, you specify either true
(prefer this style) or false
(do not prefer this style) for value
. For other rules, you specify a value such as flush_left
or before_and_after
to describe when and where to apply the rule. You don't specify a severity.
.NET formatting rules
The formatting rules in this section apply to both C# and Visual Basic.
- Organize usings
- dotnet_sort_system_directives_first
- dotnet_separate_import_directive_groups
Organize using directives
These formatting rules concern the sorting and display of using
directives and Imports
statements.
Example .editorconfig file:
dotnet_sort_system_directives_first
Property | Value |
---|---|
Option name | dotnet_sort_system_directives_first |
Applicable languages | C# and Visual Basic |
Introduced version | Visual Studio 2017 version 15.3 |
Option values | true - Sort System.* using directives alphabetically, and place them before other using directives.false - Do not place System.* using directives before other using directives. |
Code examples:
dotnet_separate_import_directive_groups
Property | Value |
---|---|
Option name | dotnet_separate_import_directive_groups |
Applicable languages | C# and Visual Basic |
Introduced version | Visual Studio 2017 version 15.5 |
Option values | true - Place a blank line between using directive groups.false - Do not place a blank line between using directive groups. |
Code examples:
C# formatting rules
The formatting rules in this section apply only to C# code.
- Newline options
- csharp_new_line_before_open_brace
- csharp_new_line_before_else
- csharp_new_line_before_catch
- csharp_new_line_before_finally
- csharp_new_line_before_members_in_object_initializers
- csharp_new_line_before_members_in_anonymous_types
- csharp_new_line_between_query_expression_clauses
- Indentation options
- csharp_indent_case_contents
- csharp_indent_switch_labels
- csharp_indent_labels
- csharp_indent_block_contents
- csharp_indent_braces
- csharp_indent_case_contents_when_block
- Spacing options
- csharp_space_after_cast
- csharp_space_after_keywords_in_control_flow_statements
- csharp_space_between_parentheses
- csharp_space_before_colon_in_inheritance_clause
- csharp_space_after_colon_in_inheritance_clause
- csharp_space_around_binary_operators
- csharp_space_between_method_declaration_parameter_list_parentheses
- csharp_space_between_method_declaration_empty_parameter_list_parentheses
- csharp_space_between_method_declaration_name_and_open_parenthesis
- csharp_space_between_method_call_parameter_list_parentheses
- csharp_space_between_method_call_empty_parameter_list_parentheses
- csharp_space_between_method_call_name_and_opening_parenthesis
- csharp_space_after_comma
- csharp_space_before_comma
- csharp_space_after_dot
- csharp_space_before_dot
- csharp_space_after_semicolon_in_for_statement
- csharp_space_before_semicolon_in_for_statement
- csharp_space_around_declaration_statements
- csharp_space_before_open_square_brackets
- csharp_space_between_empty_square_brackets
- csharp_space_between_square_brackets
- Wrap options
- csharp_preserve_single_line_statements
- csharp_preserve_single_line_blocks
- Using directive options
- csharp_using_directive_placement
New-line options
These formatting rules concern the use of new lines to format code.
Example .editorconfig file:
csharp_new_line_before_open_brace
This rule concerns whether an open brace {
should be placed on the same line as the preceding code, or on a new line. For this rule, you specify all, none, or one or more code elements such as methods or properties, to define when this rule should be applied. To specify multiple code elements, separate them with a comma (,).
Property | Value |
---|---|
Option name | csharp_new_line_before_open_brace |
Applicable languages | C# |
Introduced version | Visual Studio 2017 version 15.3 |
Option values | all - Require braces to be on a new line for all expressions ('Allman' style).none - Require braces to be on the same line for all expressions ('K&R').accessors , anonymous_methods , anonymous_types , control_blocks , events , indexers , lambdas , local_functions , methods , object_collection_array_initializers , properties , types - Require braces to be on a new line for the specified code element ('Allman' style). |
Code examples:
csharp_new_line_before_else
Property | Value |
---|---|
Option name | csharp_new_line_before_else |
Applicable languages | C# |
Introduced version | Visual Studio 2017 version 15.3 |
Option values | true - Place else statements on a new line.false - Place else statements on the same line. |
Microsoft Visual Studio Samples
Code examples:
csharp_new_line_before_catch
Property | Value |
---|---|
Option name | csharp_new_line_before_catch |
Applicable languages | C# |
Introduced version | Visual Studio 2017 version 15.3 |
Option values | true - Place catch statements on a new line.false - Place catch statements on the same line. |
Code examples:
csharp_new_line_before_finally
Property | Value |
---|---|
Option name | csharp_new_line_before_finally |
Applicable languages | C# |
Introduced version | Visual Studio 2017 version 15.3 |
Option values | true - Require finally statements to be on a new line after the closing brace.false - Require finally statements to be on the same line as the closing brace. |
Code examples:
csharp_new_line_before_members_in_object_initializers
Property | Value |
---|---|
Option name | csharp_new_line_before_members_in_object_initializers |
Applicable languages | C# |
Introduced version | Visual Studio 2017 version 15.3 |
Option values | true - Require members of object initializers to be on separate linesfalse - Require members of object initializers to be on the same line |
Code examples:
csharp_new_line_before_members_in_anonymous_types
Property | Value |
---|---|
Option name | csharp_new_line_before_members_in_anonymous_types |
Applicable languages | C# |
Introduced version | Visual Studio 2017 version 15.3 |
Option values | true - Require members of anonymous types to be on separate linesfalse - Require members of anonymous types to be on the same line |
Code examples:
csharp_new_line_between_query_expression_clauses
Property | Value |
---|---|
Option name | csharp_new_line_between_query_expression_clauses |
Applicable languages | C# |
Introduced version | Visual Studio 2017 version 15.3 |
Option values | true - Require elements of query expression clauses to be on separate linesfalse - Require elements of query expression clauses to be on the same line |
Code examples:
Indentation options
These formatting rules concern the use of indentation to format code.
Example .editorconfig file:
csharp_indent_case_contents
Property | Value |
---|---|
Option name | csharp_indent_case_contents |
Applicable languages | C# |
Introduced version | Visual Studio 2017 version 15.3 |
Option values | true - Indent switch case contentsfalse - Do not indent switch case contents |
- When this rule is set to true, i.
- When this rule is set to false, d.
Code examples:
csharp_indent_switch_labels
Property | Value |
---|---|
Option name | csharp_indent_switch_labels |
Applicable languages | C# |
Introduced version | Visual Studio 2017 version 15.3 |
Option values | true - Indent switch labelsfalse - Do not indent switch labels |
Code examples:
csharp_indent_labels
Property | Value |
---|---|
Option name | csharp_indent_labels |
Applicable languages | C# |
Introduced version | Visual Studio 2017 version 15.3 |
Option values | flush_left - Labels are placed at the leftmost columnone_less_than_current - Labels are placed at one less indent to the current contextno_change - Labels are placed at the same indent as the current context |
Code examples:
csharp_indent_block_contents
Property | Value |
---|---|
Option name | csharp_indent_block_contents |
Applicable languages | C# |
Option values | true - false - |
Code examples:
csharp_indent_braces
Property | Value |
---|---|
Option name | csharp_indent_braces |
Applicable languages | C# |
Option values | true - false - |
Code examples:
csharp_indent_case_contents_when_block
Property | Value |
---|---|
Option name | csharp_indent_case_contents_when_block |
Applicable languages | C# |
Option values | true - false - |
Code examples:
Spacing options
These formatting rules concern the use of space characters to format code.
Example .editorconfig file:
csharp_space_after_cast
Property | Value |
---|---|
Option name | csharp_space_after_cast |
Applicable languages | C# |
Introduced version | Visual Studio 2017 version 15.3 |
Option values | true - Place a space character between a cast and the valuefalse - Remove space between the cast and the value |
Code examples:
csharp_space_after_keywords_in_control_flow_statements
Property | Value |
---|---|
Option name | csharp_space_after_keywords_in_control_flow_statements |
Applicable languages | C# |
Introduced version | Visual Studio 2017 version 15.3 |
Option values | true - Place a space character after a keyword in a control flow statement such as a for loopfalse - Remove space after a keyword in a control flow statement such as a for loop |
Code examples:
csharp_space_between_parentheses
Property | Value |
---|---|
Option name | csharp_space_between_parentheses |
Applicable languages | C# |
Introduced version | Visual Studio 2017 version 15.3 |
Option values | control_flow_statements - Place space between parentheses of control flow statementsexpressions - Place space between parentheses of expressionstype_casts - Place space between parentheses in type casts |
If you omit this rule or use a value other than control_flow_statements
, expressions
, or type_casts
, the setting is not applied.
Code examples:
csharp_space_before_colon_in_inheritance_clause
Property | Value |
---|---|
Option name | csharp_space_before_colon_in_inheritance_clause |
Applicable languages | C# |
Introduced version | Visual Studio 2017 version 15.7 |
Option values | true - Place a space character before the colon for bases or interfaces in a type declarationfalse - Remove space before the colon for bases or interfaces in a type declaration |
Code examples: Install mojave on catalina.
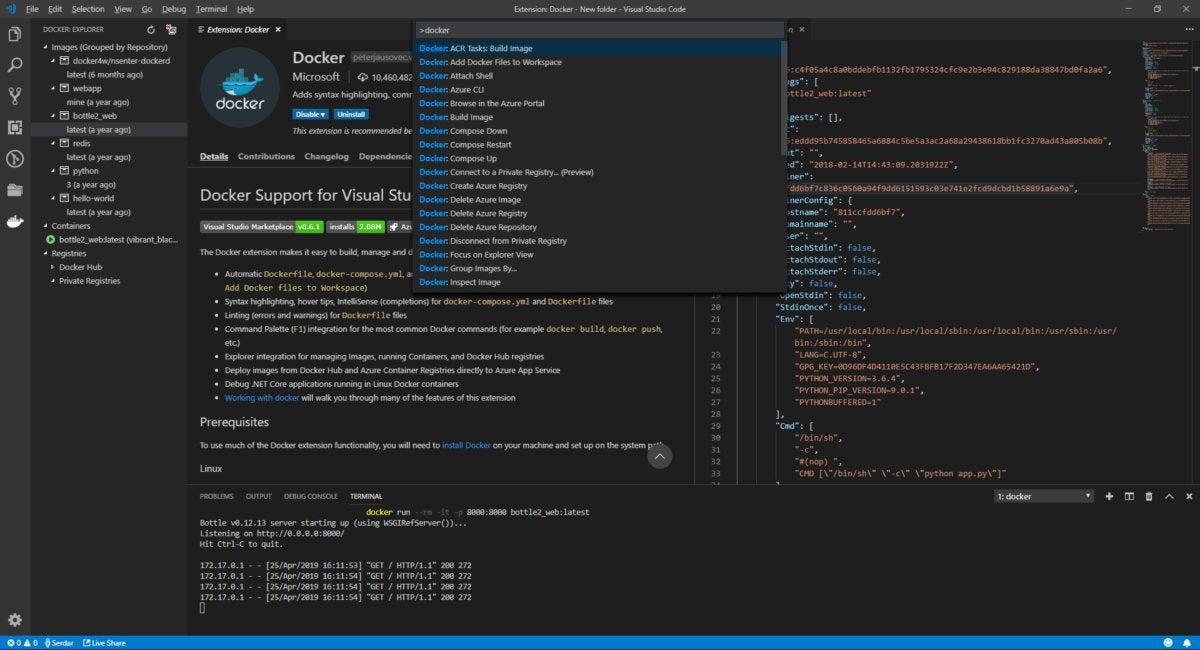
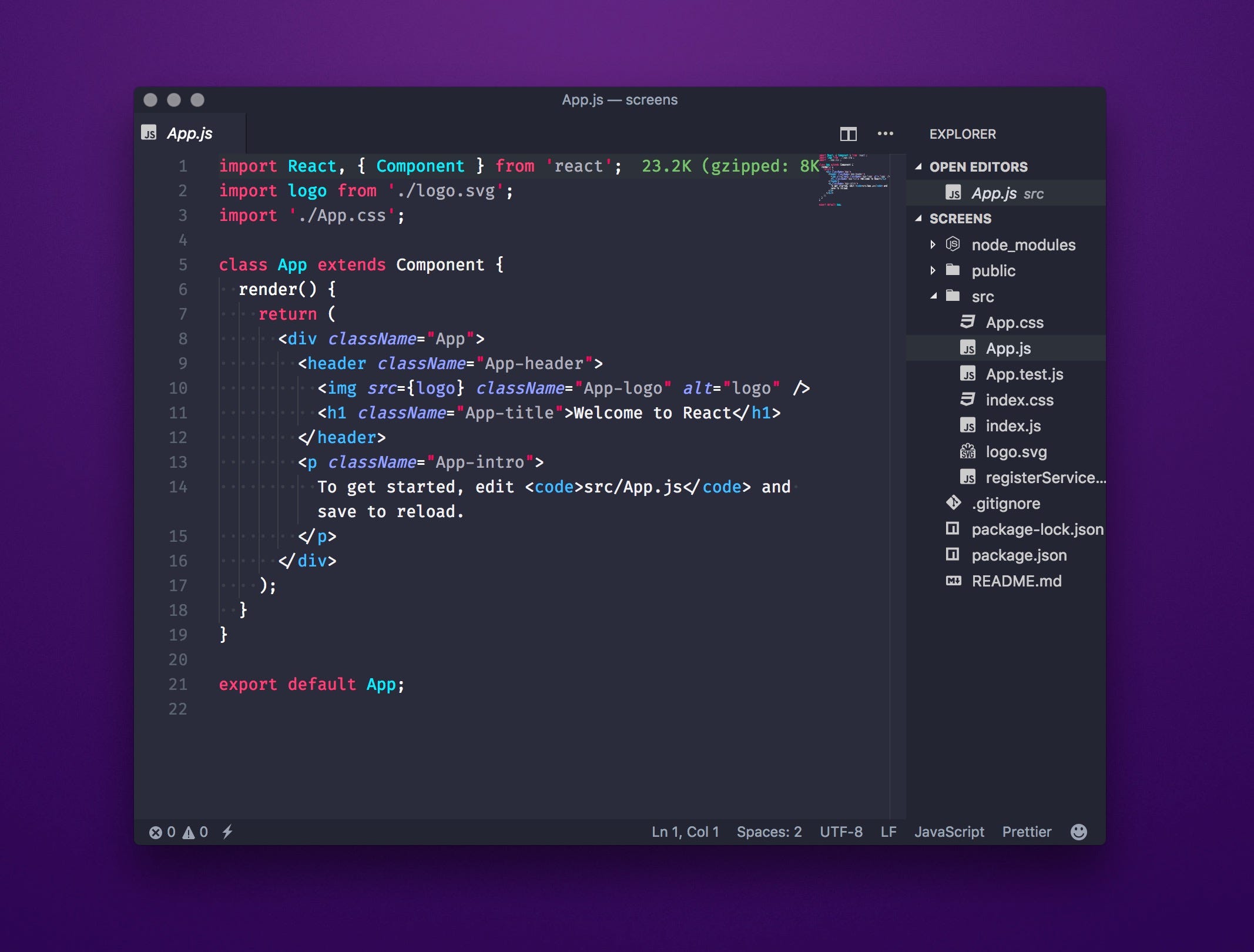
csharp_space_after_colon_in_inheritance_clause
Property | Value |
---|---|
Option name | csharp_space_after_colon_in_inheritance_clause |
Applicable languages | C# |
Introduced version | Visual Studio 2017 version 15.7 |
Option values | true - Place a space character after the colon for bases or interfaces in a type declarationfalse - Remove space after the colon for bases or interfaces in a type declaration |
Code examples:
csharp_space_around_binary_operators
Property | Value |
---|---|
Option name | csharp_space_around_binary_operators |
Applicable languages | C# |
Introduced version | Visual Studio 2017 version 15.7 |
Option values | before_and_after - Insert space before and after the binary operatornone - Remove spaces before and after the binary operatorignore - Ignore spaces around binary operators |
Visual Studio Force Formatting
If you omit this rule, or use a value other than before_and_after
, none
, or ignore
, the setting is not applied.
Code examples:
csharp_space_between_method_declaration_parameter_list_parentheses
Property | Value |
---|---|
Option name | csharp_space_between_method_declaration_parameter_list_parentheses |
Applicable languages | C# |
Introduced version | Visual Studio 2017 version 15.3 |
Option values | true - Place a space character after the opening parenthesis and before the closing parenthesis of a method declaration parameter listfalse - Remove space characters after the opening parenthesis and before the closing parenthesis of a method declaration parameter list |
Code examples:
csharp_space_between_method_declaration_empty_parameter_list_parentheses
Property | Value |
---|---|
Option name | csharp_space_between_method_declaration_empty_parameter_list_parentheses |
Applicable languages | C# |
Introduced version | Visual Studio 2017 version 15.7 |
Option values | true - Insert space within empty parameter list parentheses for a method declarationfalse - Remove space within empty parameter list parentheses for a method declaration |
Code examples:
csharp_space_between_method_declaration_name_and_open_parenthesis
Property | Value |
---|---|
Option name | csharp_space_between_method_declaration_name_and_open_parenthesis |
Applicable languages | C# |
Option values | true - Place a space character between the method name and opening parenthesis in the method declarationfalse - Remove space characters between the method name and opening parenthesis in the method declaration |
Code examples:
csharp_space_between_method_call_parameter_list_parentheses
Property | Value |
---|---|
Option name | csharp_space_between_method_call_parameter_list_parentheses |
Applicable languages | C# |
Introduced version | Visual Studio 2017 version 15.3 |
Option values | true - Place a space character after the opening parenthesis and before the closing parenthesis of a method callfalse - Remove space characters after the opening parenthesis and before the closing parenthesis of a method call |
Code examples:
csharp_space_between_method_call_empty_parameter_list_parentheses
Property | Value |
---|---|
Option name | csharp_space_between_method_call_empty_parameter_list_parentheses |
Applicable languages | C# |
Introduced version | Visual Studio 2017 version 15.7 |
Option values | true - Insert space within empty argument list parenthesesfalse - Remove space within empty argument list parentheses |
Code examples:
csharp_space_between_method_call_name_and_opening_parenthesis
Property | Value |
---|---|
Option name | csharp_space_between_method_call_name_and_opening_parenthesis |
Applicable languages | C# |
Introduced version | Visual Studio 2017 version 15.7 |
Option values | true - Insert space between method call name and opening parenthesisfalse - Remove space between method call name and opening parenthesis |
Code examples:
csharp_space_after_comma
Property | Value |
---|---|
Option name | csharp_space_after_comma |
Applicable languages | C# |
Option values | true - Insert space after a commafalse - Remove space after a comma |
Code examples:
csharp_space_before_comma
Property | Value |
---|---|
Option name | csharp_space_before_comma |
Applicable languages | C# |
Option values | true - Insert space before a commafalse - Remove space before a comma |
Code examples:
csharp_space_after_dot
Property | Value |
---|---|
Option name | csharp_space_after_dot |
Applicable languages | C# |
Option values | true - Insert space after a dotfalse - Remove space after a dot |
Code examples:
csharp_space_before_dot
Property | Value |
---|---|
Option name | csharp_space_before_dot |
Applicable languages | C# |
Option values | true - Insert space before a dot false - Remove space before a dot |
Code examples:
csharp_space_after_semicolon_in_for_statement
Property | Value |
---|---|
Option name | csharp_space_after_semicolon_in_for_statement |
Applicable languages | C# |
Option values | true - Insert space after each semicolon in a for statementfalse - Remove space after each semicolon in a for statement |
Code examples:
csharp_space_before_semicolon_in_for_statement
Property | Value |
---|---|
Option name | csharp_space_before_semicolon_in_for_statement |
Applicable languages | C# |
Option values | true - Insert space before each semicolon in a for statement false - Remove space before each semicolon in a for statement |
Code examples:
csharp_space_around_declaration_statements
Property | Value |
---|---|
Option name | csharp_space_around_declaration_statements |
Applicable languages | C# |
Option values | ignore - Don't remove extra space characters in declaration statementsfalse - Remove extra space characters in declaration statements |
Microsoft Visual Studio Guide
Code examples:
csharp_space_before_open_square_brackets
Property | Value |
---|---|
Option name | csharp_space_before_open_square_brackets |
Applicable languages | C# |
Option values | true - Insert space before opening square brackets [ false - Remove space before opening square brackets [ |
Code examples:
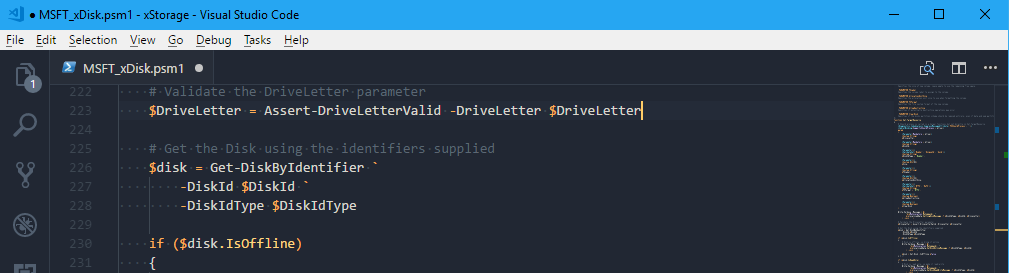
csharp_space_between_empty_square_brackets
Property | Value |
---|---|
Option name | csharp_space_between_empty_square_brackets |
Applicable languages | C# |
Option values | true - Insert space between empty square brackets [ ] false - Remove space between empty square brackets [] |
Code examples:
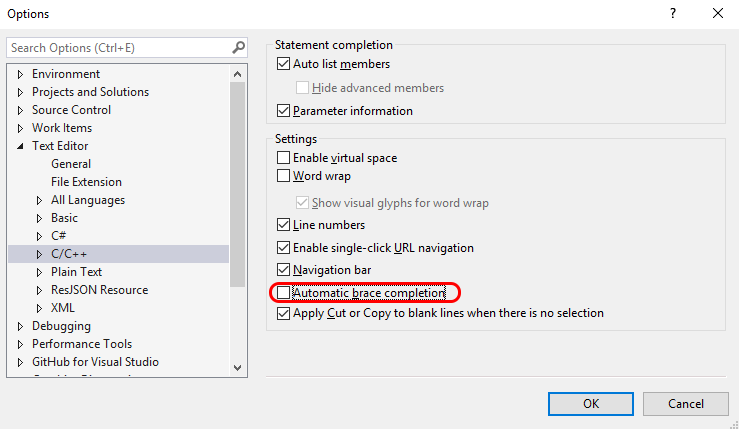
csharp_space_between_square_brackets
Property | Value |
---|---|
Option name | csharp_space_between_square_brackets |
Applicable languages | C# |
Option values | true - Insert space characters in non-empty square brackets [ 0 ] false - Remove space characters in non-empty square brackets [0] |
Code examples:
Visual Studio Formatting Settings
Wrap options
These formatting rules concern the use of single lines versus separate lines for statements and code blocks.
Example .editorconfig file:
csharp_preserve_single_line_statements
Property | Value |
---|---|
Option name | csharp_preserve_single_line_statements |
Applicable languages | C# |
Introduced version | Visual Studio 2017 version 15.3 |
Option values | true - Leave statements and member declarations on the same linefalse - Leave statements and member declarations on different lines |
Code examples:
csharp_preserve_single_line_blocks
Property | Value |
---|---|
Option name | csharp_preserve_single_line_blocks |
Applicable languages | C# |
Introduced version | Visual Studio 2017 version 15.3 |
Option values | true - Leave code block on single linefalse - Leave code block on separate lines |
Code examples:
Using directive options
This formatting rule concerns the use of using directives being placed inside versus outside a namespace.
Visual Studio 2017 Formatting
Example .editorconfig file:
csharp_using_directive_placement
Property | Value |
---|---|
Option name | csharp_using_directive_placement |
Applicable languages | C# |
Introduced version | Visual Studio 2019 version 16.1 |
Option values | outside_namespace - Leave using directives outside namespaceinside_namespace - Leave using directives inside namespace |
Code examples:
See also
